CSS Interview Questions
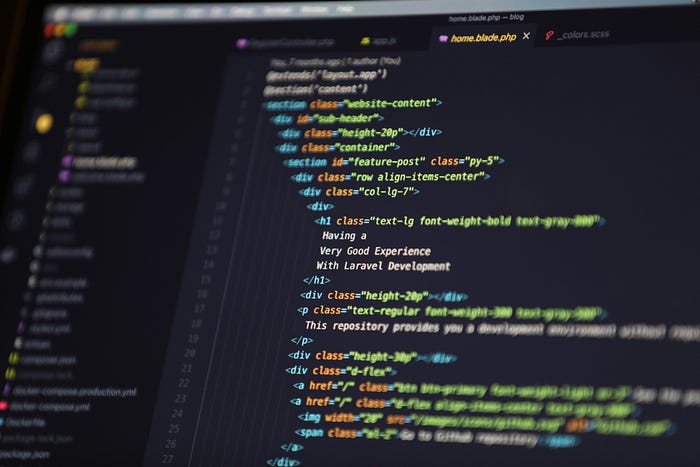
What is the CSS Box Model, and what are its components?
The CSS Box Model is a layout concept defining the structure of elements on a webpage. It includes:
- Content: The actual material like text or images.
- Padding: Space between content and border.
- Border: Surrounds the padding, customizable in color, style, and thickness.
- Margin: External space around the border separating elements.
* box-sizing: border-box; : It includes padding and border in the element’s total width and height, making layout sizing more predictable.
What is the difference between `static`, `relative`, `absolute`, `fixed`, and `sticky` positioning in CSS?
static: Default. Elements flow normally in the document.
relative: Moves from its normal position using top, left, etc.
absolute: Positions out of normal flow relative to the nearest positioned ancestor, using top, left, etc.
fixed: Similar to absolute but relative to the viewport and remains fixed during scrolling.
sticky: Acts like relative until a scroll threshold is reached, then behaves like fixed.
Usage:
- relative for minor adjustments.
- absolute or fixed for precise positioning.
- sticky for elements that need to stay visible during scroll.
What is Flexbox, and explain it’s properties?
Flexbox, or the Flexible Box Model, is a CSS layout tool that simplifies creating flexible and responsive layouts. It aligns and distributes space among items within a container, even when their size is unknown.
Container Properties
- `display: flex;` — Defines a flex container.
- `flex-direction` — Sets row or column flow.
- `flex-wrap` — Controls item wrapping.
- `justify-content` — Aligns items horizontally.
- `align-items` — Aligns items vertically.
- `align-content` — Manages space between rows.
- `gap` — Adds space between items within a flex container.
Item Properties
- `flex-grow` — Allows item to grow.
- `flex-shrink` — Allows item to shrink.
- `flex-basis` — Sets base size.
- `flex` — Shorthand for grow, shrink, and basis.
- `align-self` — Overrides vertical alignment.
What are Grids, and explain it’s properties?
CSS Grid is a layout system in CSS designed for building two-dimensional layouts, ideal for managing both columns and rows in web designs. Here’s a brief overview of its key properties and an example:
Key Properties
- display: grid: Activates the grid layout on an element.
- grid-template-columns / grid-template-rows: Defines the columns and rows of the grid.
- grid-gap: Sets the space between grid items.
CSS Grid vs. Flexbox
CSS Grid:
- Designed for: Two-dimensional layouts (rows and columns).
- Use when: You need control over complex arrangements and precise alignment across both dimensions, like page layouts or advanced grid patterns.
Flexbox:
- Designed for: One-dimensional layouts (either a row or a column).
- Use when: You require flexibility for items within a linear axis, ideal for components like menus or simple stacks of elements adjusting dynamically.
Choosing Between Them:
- Use Grid for complex structures requiring alignment in both rows and columns.
- Use Flexbox for simpler, flexible alignments primarily along a single axis.
What are pseudo-classes and pseudo-elements in CSS?
- Pseudo-classes: They are used to define the special state of an element (e.g., `:hover`, `:focus`, `:nth-child`).
- Pseudo-elements: They are used to style specific parts of an element (e.g., `::before`, `::after`, `::first-letter`).
What are CSS selectors, and what are some commonly used ones?
CSS selectors are patterns used to select and style HTML elements. Commonly used selectors include:
- Universal selector (`*`): Selects all elements.
- Type selector (`div`): Selects all elements of a specific type.
- Class selector (`.className`): Selects elements with a specific class.
- ID selector (`#idName`): Selects an element with a specific ID.
- Attribute selector (`[type=”text”]`): Selects elements with a specific attribute.
What is Specificity in CSS? How to Adjust it?
Specificity in CSS determines which style rules are applied to an element when multiple rules conflict. It’s calculated based on the type of selectors used in a rule:
- Inline styles (within the HTML) have the highest specificity.
- IDs are next in priority.
- Classes, attributes, and pseudo-classes follow.
- Elements and pseudo-elements have the lowest specificity.
To increase specificity:
- Add more IDs or classes to a selector.
- Use inline styles directly on the element.
To decrease specificity:
- Use more generic selectors like element types.
#header {
color: blue;
} /* Specificity: 0,1,0,0 */
div#header {
color: green;
} /* Specificity: 0,1,0,1 */
.header {
color: red;
} /* Specificity: 0,0,1,0 */
div.header {
color: orange;
} /* Specificity: 0,0,1,1 */
Here, `div#header` will override `#header` due to its higher specificity (more elements involved).
What is the difference between `display: none` and `visibility: hidden`?
- `display: none`: Completely removes the element from the document flow, and it will not occupy any space.
- `visibility: hidden`: Makes the element invisible, but it still occupies the space in the layout.
How can you center a div?
Flexbox:
.parent {
display: flex;
justify-content: center; /* Horizontal centering */
align-items: center; /* Vertical centering */
}
Grid:
.parent {
display: grid;
place-items: center;
}
Absolute Positioning with Transform:
.center {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Difference between em and rem units in CSS
em: Relative to the font size of the nearest parent element; can compound in nested elements.
rem: Relative to the root element (<html>) font size; consistent across the document.
Use em for flexible, context-based scaling.
Use rem for consistent, document-wide scaling.
What are CSS Mixins?
CSS mixins are a feature of CSS preprocessors like Sass and Less, allowing for reusable sets of CSS properties. They help streamline your CSS by letting you define styles once and reuse them throughout your stylesheets with parameters to customize their application.
@mixin box-shadow($x, $y, $blur, $color) {
box-shadow: $x $y $blur $color;
}
.box {
@include box-shadow(0px, 0px, 10px, rgba(0, 0, 0, 0.5));
}
Benefits:
- Reusability: Avoid repeating CSS code.
- Maintainability: Update in one place to affect all uses.
- Readability: Keeps stylesheets clean and organised.
What are media queries and why are they important?
Media queries are a feature of CSS that enable web content to adapt to different conditions like screen sizes or orientations. They are important for creating responsive designs and ensuring a website looks good on all devices.
/* Base styles */
body {
background-color: lightblue;
font-size: 18px;
color: navy;
}
/* Media query for screens wider than 600px */
@media (min-width: 600px) {
body {
background-color: peachpuff;
font-size: 20px;
color: darkred;
}
}
How do you create animations in CSS?
You create animations in CSS using the @keyframes rule to define the animation sequence and the animation properties to control the timing, duration, and other aspects of the animation.
Bouncing Ball
@keyframes bounce {
0%,
100% {
transform: translateY(0);
animation-timing-function: ease-in;
}
50% {
transform: translateY(-20px);
animation-timing-function: ease-out;
}
}
.ball {
width: 50px;
height: 50px;
background-color: red;
border-radius: 50%;
animation: bounce 1s infinite;
}
What are some techniques for optimizing CSS performance?
- Minimize CSS: Reduce file size by removing unused CSS and compressing the files.
- Simplify Selectors: Use efficient, straightforward selectors to speed up rendering.
- Use CSS Sprites: Combine multiple images into a single image to reduce HTTP requests.
- Leverage Browser Caching: Use server settings to allow browsers to store CSS files, reducing load times for repeat visitors.